How can wordpress connect to ChatGPT? Do you have some example’s code?
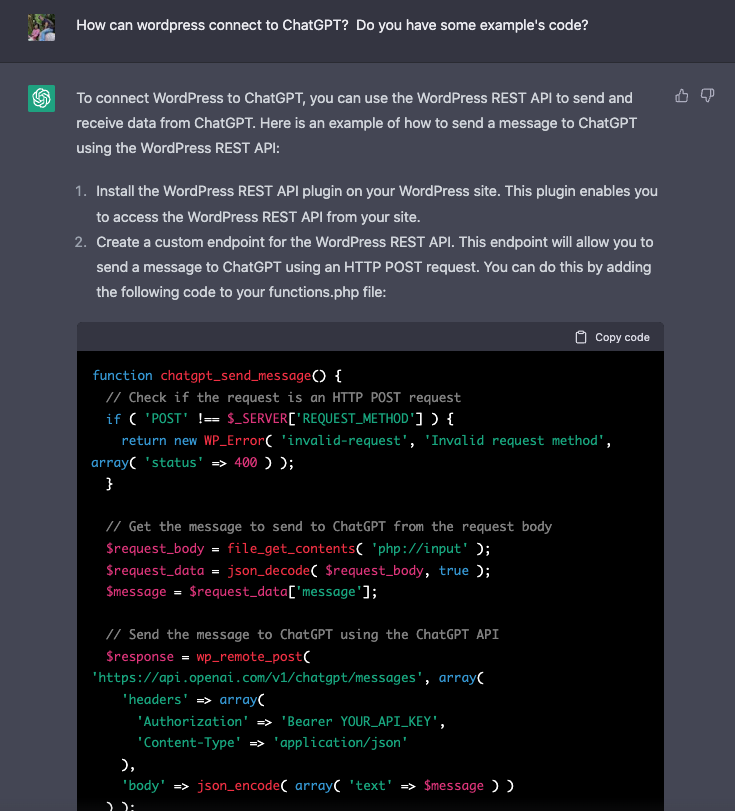
To connect WordPress to ChatGPT, you can use the WordPress REST API to send and receive data from ChatGPT. Here is an example of how to send a message to ChatGPT using the WordPress REST API:
- Install the WordPress REST API plugin on your WordPress site. This plugin enables you to access the WordPress REST API from your site.
- Create a custom endpoint for the WordPress REST API. This endpoint will allow you to send a message to ChatGPT using an HTTP POST request. You can do this by adding the following code to your functions.php file:
function chatgpt_send_message() {
// Check if the request is an HTTP POST request
if ( 'POST' !== $_SERVER['REQUEST_METHOD'] ) {
return new WP_Error( 'invalid-request', 'Invalid request method', array( 'status' => 400 ) );
}
// Get the message to send to ChatGPT from the request body
$request_body = file_get_contents( 'php://input' );
$request_data = json_decode( $request_body, true );
$message = $request_data['message'];
// Send the message to ChatGPT using the ChatGPT API
$response = wp_remote_post( 'https://api.openai.com/v1/chatgpt/messages', array(
'headers' => array(
'Authorization' => 'Bearer YOUR_API_KEY',
'Content-Type' => 'application/json'
),
'body' => json_encode( array( 'text' => $message ) )
) );
// Return the response from ChatGPT
return $response['body'];
}
// Register the custom endpoint for the WordPress REST API
add_action( 'rest_api_init', function() {
register_rest_route( 'chatgpt/v1', '/send-message', array(
'methods' => 'POST',
'callback' => 'chatgpt_send_message'
) );
} );
- Test the custom endpoint by sending an HTTP POST request to the endpoint with a message in the request body. For example, you can use the following curl command to send a message to ChatGPT:
curl -X POST -H "Content-Type: application/json" -d '{"message":"Hello ChatGPT!"}' https://example.com/wp-json/chatgpt/v1/send-message
This will send the message “Hello ChatGPT!” to ChatGPT, and the response from ChatGPT will be returned in the response from the custom endpoint.
You can use this approach to send and receive messages from ChatGPT in your WordPress site by calling the custom endpoint from your WordPress code or from an external application.
ถามว่ามี Code เป็น Python’s Flask Platform API ไหม? มีด้วย
do you have this code in python’s flask platform API?
Yes, here is an example of how to implement the ChatGPT API in a Python Flask API:
- Install the Flask and Requests libraries:
pip install flask requests
- Create a Flask API with a route for sending messages to ChatGPT:
from flask import Flask, request
import requests
app = Flask(__name__)
@app.route('/send-message', methods=['POST'])
def send_message():
# Get the message to send to ChatGPT from the request body
message = request.json['message']
# Send the message to ChatGPT using the ChatGPT API
response = requests.post('https://api.openai.com/v1/chatgpt/messages',
headers={
'Authorization': 'Bearer YOUR_API_KEY',
'Content-Type': 'application/json'
},
json={'text': message}
)
# Return the response from ChatGPT
return response.text
if __name__ == '__main__':
app.run()
- Run the Flask API:
python app.py
- Test the API by sending an HTTP POST request to the /send-message endpoint with a message in the request body. For example, you can use the following curl command to send a message to ChatGPT:
curl -X POST -H "Content-Type: application/json" -d '{"message":"Hello ChatGPT!"}' http://localhost:5000/send-message
This will send the message “Hello ChatGPT!” to ChatGPT, and the response from ChatGPT will be returned in the response from the Flask API.
You can use this approach to send and receive messages from ChatGPT in your Python application by calling the Flask API route from your code or from an external application.